Console Logs are very important in any client-side application debugging.
It helps us (developers) to show an important information for debugging. We use it to debug the state of variables, showing warning messages, error messages, and some informational messages.
Generally, When you develop an application there are so many logs generated on a console. Also, multiple developers work on a single application.
So it is important to write logs in a proper format, so each developer can understand it. otherwise, it becomes difficult to identify the importance of each log.
Keeping this in mind, Recently I have published🚀 the Angular Console Logging Utility: NGX-FANCY-LOGGER
So before I describe the features of this library let me tell you real-world scenarios which I faced with logging using normal console.log()
, which gives me an idea to create an angular console logging utility.
Angular Console Logging Utility
npm i ngx-fancy-logger
Make debugging easier with more readable and configurable console logs.
- Different Log Level Labels – Identifying important Logs
- It is very difficult to loop through all logs and find important logs.
- Solution: for this purpose, I have added labels in NGX-FANCY-LOGGER, this will make clear to understand important and informational logs.
- Environment Specific Logs
-
- Generally, some logs we only want to show on the development environment and only error and warning logs we want to show on the production environment, this is not possible with normal
console.log()
statements. - Solution: NGX-FANCY-LOGGER has a feature to set Environment Specific
logLevel
.
- Generally, some logs we only want to show on the development environment and only error and warning logs we want to show on the production environment, this is not possible with normal
-
- Highlight important message as Headers
- Solution: We can generate a kind of header on the console using NGX-FANCY-LOGGER.
- Disable All Logs on Console
- Sometimes we don’t want to show any logs on console.
- Solution: NGX-FANCY-LOGGER has a feature to disable all logs generated with its logger method.
- Debugging RxJS Observable Stream
- Many times we need to debug RxJS Observable Data Streams: we generally do this with
tap(data=> console.log(data))
, putting this at each place is a very tedious task. - Solution: To resolve such type of scenario NGX-FANCY-LOGGER has
debugOperator()
operator function. You just need to add this operator, the way we use other RxJS operators, in this operator you can also specifylogLevel
andmessage
.
- Many times we need to debug RxJS Observable Data Streams: we generally do this with
- Different Log Levels (INFO=0, DEBUG=1, WARNING=2, ERROR=3).
- Log Levels are displayed in Label form with assigned color style or default colors.
- Show/Hide Time for each Log Level
- Show/Hide Emoji for each Log Level
- Log Level colors and emojis are configurable
- Show Header on console (
color
andfontSize
configurable) - Debug RxJS Observable Stream using
debugOperator()
operator function - Can configure each setting with
LoggerConfig
inforRoot
(which allows us to configure environment-specific configuration) or usingupdateConfig()
method. - Reset configuration using
resetConfig()
method - Environment Specific Log Level Restriction. eg. if you set
logLevel
to WARNING, it will only show logs for WARNING and ERROR. - Can Disable all logs
Read more about NGX-FANCY-LOGGER at its official docs.
Install NGX-FANCY-LOGGER using the following command:
npm install --save ngx-fancy-logger
Once it is installed import NgxFancyLoggerModule
in AppModule
.
Use with the default configuration
To use NgxFancyLoggerModule
with the Default configuration, you just need to import it in AppModule
. No need to call .forRoot()
method.
import { NgxFancyLoggerModule } from 'ngx-fancy-logger'; @NgModule({ ... imports: [ ... NgxFancyLoggerModule ] ... }) export class AppModule { }
Override Configuration / Environment Specific Configuration
You can override NgxFancyLoggerModule
default configuration with forRoot
method, as below. Each configuration is optional.
Overriding default configuration allows us to set environment specific configuration. We can add loggerConfig
in environment.ts
(environment specific file) and use it as NgxFancyLoggerModule.forRoot(environment.loggerConfig)
in AppModule
.
import { NgxFancyLoggerModule, LogLevel } from 'ngx-fancy-logger'; @NgModule({ ... imports: [ ... NgxFancyLoggerModule.forRoot({ showTime: false, logLevel: LogLevel.WARNING, levelColor: { [LogLevel.ERROR]: 'brown' } }) ] }) export class AppModule { }
Once the installation is done we can use NgxLoggerService
in any component or service.
import { Component } from '@angular/core'; import { NgxFancyLoggerService } from 'ngx-fancy-logger'; @Component({ selector: 'app-root', templateUrl: './app.component.html', styleUrls: ['./app.component.css'] }) export class AppComponent { constructor(private logger: NgxFancyLoggerService){ logger.header('App Component Loaded...') } }
Show Different Log Levels and Header Log
export class AppComponent { ... showHeaderLog(){ this.logger.header('NgxFancyLogger Header Log with configured color and fontSize', {color: 'red', fontSize: 30}) } showDifferentLogLevels(){ this.logger.info('This is a info log', {data: {a: 20, b:30 }}); this.logger.debug('This is a debug log', {data: {a: 20, b:30 }}); this.logger.warning('This is a warning log', {data: {a: 20, b:30 }}); this.logger.error('This is a error log', {data: {a: 20, b:30 }}); } }
<button class="btn btn-primary" (click)="showHeaderLog()">Show Header Log</button> <button class="btn btn-primary" (click)="showDifferentLogLevels()">Show Different Log Levels</button>
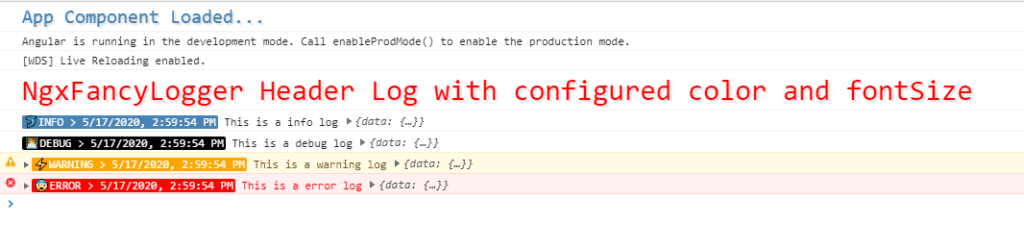
Debug RxJS Observable Stream with debugOperator()
export class AppComponent { ... debugRxJSStream(){ let source$ = interval(1000); source$.pipe( this.logger.debugOperator(), map<number, number>(data => data*100), this.logger.debugOperator('Result', LogLevel.INFO), take(5), ).subscribe(); } }
<button class="btn btn-primary" (click)="debugRxJSStream()">Debug RxJS Stream</button>
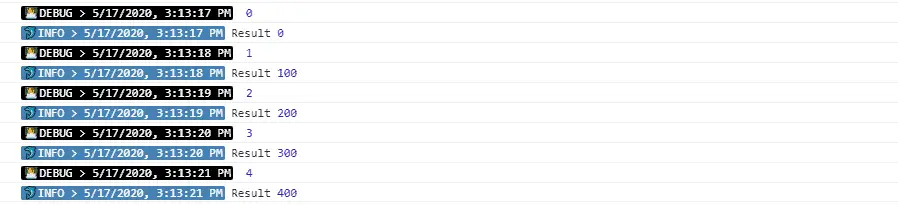
All are welcome to contribute to NGX-FANCY-LOGGER. Contribute with some code, file a bug or improve the documentation.
https://github.com/ngdevelop-tech/ngx-fancy-logger
If you like this library, mark a star ⭐ GitHub repository
In this article, we have seen how to we can make debugging easier with configurable and more readable logs generated with angular console logging utility: NGX-FANCY-LOGGER.
I hope you like this article, please provide your valuable feedback and suggestions in below comment section🙂.
For more updates, Follow us 👍 on NgDevelop Facebook page.