Google Analytics is a popular analytical tool for web and mobile applications from Google. It helps to track real-time and historical website traffic stats.
Key features :
- Tracking web site traffic, page views, sessions, bounce rate.
- Tracking traffic sources, events, conversations, referrals.
- Provides information about new users and returning users details, page load time.
- Provides information about user demographics, device type, mobile device screen resolution, language, browser and OS details, network details
- User acquisition details, Search console details like search queries, landing pages.
- Campaign details, Traffic through Google Ads, social media traffic.
and many more things. It provides reports of each above feature, which helps to analyze your users and take further business decisions to increase the growth of the product, website, or app.
Google Analytics data also helps to do SEO (Search Engine Optimization) of web and mobile apps.
Google analytics provides a tracking code snippet, which we need to put in the HEAD
section of each page. When that page is loaded it will trigger an event to capture page view.
This approach works well for traditional web applications because for each page view tracking code snippet will be loaded and executed.
However, In Single Page Application (SPA) has a single index.html
where all other routes are dynamically rendered, because of that that tracking code snippet will be loaded only once. So that google analytics event will be triggered only once when the initial page is rendered.
So in angular, to capture different route change we need manually trigger page view events.
So In this article, we will see step by step implementation of google analytics with angular application.
In this article, we will not cover angular application development from scratch,
I have created one angular application which has two routes /home
and /demo
.
We will integrate google analytics in this application to capture page views.
We will require the google analytics tracking code for our application. So let’s get it.
- Sign in to Google Analytics
- Open Admin Panel and click on + Create Property.
A property represents your website or app, and is the collection point in Analytics for the data from your site or app. You can create multiple properties with one google analytics account.
- Select Web or App and fill the required details – Website name, website URL, Industry Category and TimeZone
- Now click on the create button to create a property.
Once your property is created it will generate global site tag(gtag.js) snippet for your application.
<!-- Global site tag (gtag.js) - Google Analytics --> <script async src="https://www.googletagmanager.com/gtag/js?id=TRACKING_CODE"></script> <script> window.dataLayer = window.dataLayer || []; function gtag(){dataLayer.push(arguments);} gtag('js', new Date()); gtag('config',[TRACKING_CODE]); </script>
Note : TRACKING_CODE
is your application’s unique tracking code, it will be like UA-XXXXX-X
Now, above tracking code snippet we will install in our application to track page views.
Copy and paste the tracking code in head
section of index.html
as shown below
<!doctype html> <html lang="en"> <head> ... ... <!-- Global site tag (gtag.js) - Google Analytics --> <script async src="https://www.googletagmanager.com/gtag/js?id=TRACKING-CODE"></script> <script> window.dataLayer = window.dataLayer || []; function gtag() { dataLayer.push(arguments); } gtag('js', new Date()); /** Disable automatic page view hit to fix duplicate page view count **/ gtag('config', 'TRACKING-CODE', {send_page_view: false}); </script> </head> <body> <app-root>App Loading...</app-root> </body> </html>
Replace TRACKING-CODE with your tracking code. it will be like UA-XXXXX-X.
Note:
gtag(config, TRACKING-CODE)
trigger’s an event to capture page view, but in our app, we will capture page views on route change.
So REPLACE gtag(config, TRACKING-CODE)
with gtag('config', 'TRACKING-CODE', { send_page_view: false});
So that it is doesn’t count page view twice.
Here, We want to trigger the google analytics page view event on route change.
For this, we need to subscribe to the Router.events
and on NavigationEnd
event we will trigger the google analytics page view event.
We will add router event subscription in app.component.ts
. You can also create separate service to handle google analytics page views and events.
We will use gtag
function which is globally exported by gtag.js
to trigger a google analytics page view event.
... import { Router, NavigationEnd } from '@angular/router'; import { filter } from 'rxjs/operators'; declare const gtag: Function; @Component({ selector: 'app-root', templateUrl: './app.component.html', styleUrls: ['./app.component.css'] }) export class AppComponent { ... constructor(private router: Router) { ... this.router.events.pipe( filter(event => event instanceof NavigationEnd) ).subscribe((event: NavigationEnd) => { /** START : Code to Track Page View */ gtag('event', 'page_view', { page_path: event.urlAfterRedirects }) /** END */ }) } }
Great ✨✨✨ We are done with the google analytics setup in angular.
Now open google analytics and test whether it is capturing page views properly or not.
As you can see below, Google Analytics is now showing the number of users and active pages.
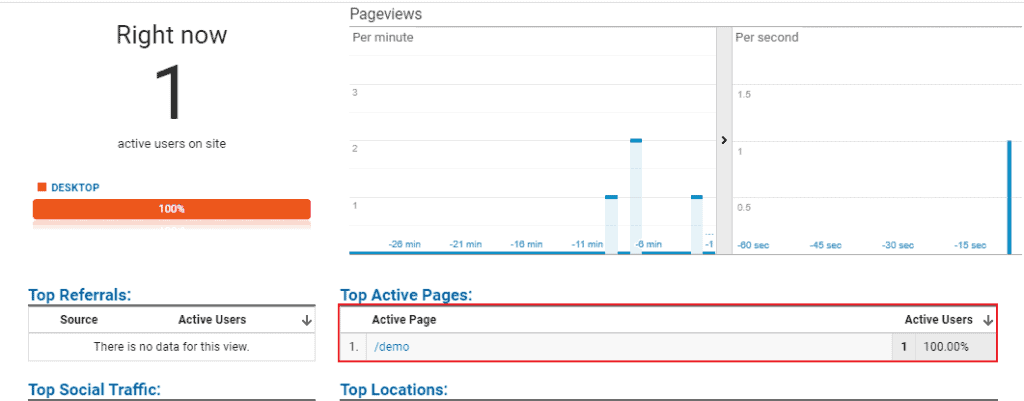
Sometimes, We required to use environment-specific google analytics tracking id.
For example, For the development and production environment we want to use separate google analytics tracking-id.
In this scenario, we have to dynamically configure google analytics tracking ID.
Follow the below steps to implement it:
- Remove external Google Tag Manager (gtag/js) script from
index.html
Because we are required to pass the tracking id in this script, We will add this script dynamically. - Remove
gtag('config', 'TRACKING-ID', { send_page_view: false }
from the following script inindex.html
<script> window.dataLayer = window.dataLayer || []; function gtag(){dataLayer.push(arguments);} gtag('js', new Date()); </script>
- Set
GA_TRACKING_ID
property with your Google Analytics Tracking ID inenvironment.ts
andenvironment.prod.ts
export const environment = { ... GA_TRACKING_ID: 'YOUR-GA-TRACKING-ID' };
- Dynamically add Google Tag Manager Script
Now inapp.component.ts
file we will create one functionaddGAScript()
to add google analytics (google tag manager) script dynamically. here we will set the tracking id insrc
URL from the environment variable. We will call this function in constructor ofapp.component.ts
import { Component } from '@angular/core'; import { Router, NavigationEnd } from '@angular/router'; import { filter } from 'rxjs/operators'; import { environment } from '../environments/environment'; declare const gtag: Function; @Component({ selector: 'app-root', templateUrl: './app.component.html', styleUrls: ['./app.component.css'] }) export class AppComponent { ... constructor(private router: Router) { ... this.addGAScript(); this.router.events.pipe( filter(event => event instanceof NavigationEnd) ).subscribe((event: NavigationEnd) => { /** START : Code to Track Page View */ gtag('event', 'page_view', { page_path: event.urlAfterRedirects }) /** END */ }) } /** Add Google Analytics Script Dynamically */ addGAScript() { let gtagScript: HTMLScriptElement = document.createElement('script'); gtagScript.async = true; gtagScript.src = 'https://www.googletagmanager.com/gtag/js?id=' + environment.GA_TRACKING_ID; document.head.prepend(gtagScript); /** Disable automatic page view hit to fix duplicate page view count **/ gtag('config', environment.GA_TRACKING_ID, { send_page_view: false }); } }
As you can see in the above code snippet, we are setting google analytics tracking id from environment variable GA_TRACKING_ID.
Great ✨✨✨ We are done with the Environment Specific configuration for google analytics in angular. Now test it in Google Analytics Dashboard.
If you find any issue in configuration let me know in the comment section. If it works for you, please give a like and share this article with others for more reach😊.
In this article, we have seen the integration of Google Analytics with Angular. We have also configured the environment-specific google analytics configuration.
I hope you like this article, please provide your valuable feedback and suggestions in comment section below🙂.
For more updates, Follow us 👍 on NgDevelop Facebook page.