Angular Pipes
Angular Pipes are used to transform data on a template, without writing a boilerplate code in a component.
A pipe takes in data as input and transforms it to the desired output. It is like a filter in Angular 1 (AngularJS).
Generally, If we need to transform data, we write the code in the component, For example, we want to transform today’s date into a format like '16 Apr 2018'
or '16-04-2018'
, We need to write separate code in the component.
So instead of writing separate boilerplate code, we can use the built-in pipe called DatePipe
which will take input and transform it into the desired date format.
We can use the pipe on a template using Pipe Operator |
.
As shown below,
{{today | date : ‘fullDate’}}
This interpolation give output in Monday, April 16, 2018
date format.
here,
today
is the component variable, which specifies the current date.date
represent DataPipefullDate
is an optional parameter or argument which specifies the date format.
DatePipe
, UpperCasePipe
, LowerCasePipe
. Other than this, We can also create our own custom pipe.Angular comes with a collection of built-in pipes such as DatePipe
, UpperCasePipe
, LowerCasePipe
, CurrencyPipe
, DecimalPipe
, and PercentPipe
. They are all available for use in any template.
Built-in Angular Pipes are defined in @angular/common
package.
Let’s see each pipe with an example.
1. UpperCasePipe
UpperCasePipe transforms text to uppercase.
We can use UpperCasePipe as shown below,
expression | uppercase
here,
expression
is any string valueuppercase
represent UpperCasePipe
Example :
<div class="card"> <div class="card-body"> <h4 class="card-title">Upper Case Pipe</h4> <p ngNonBindable>{{'Welcome to Angular Pipes' | uppercase}}</p> <p>{{'Welcome to Angular Pipes' | uppercase}}</p> </div> </div>
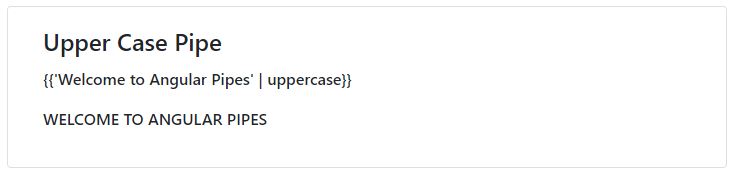
2. LowerCasePipe
As the name suggests, LowerCasePipe transforms text to lowercase.
We can use LowerCasePipe as shown below,
expression | lowercase
here,
expression
is any string valuelowercase
represent LowerCasePipe
Example :
<div class="card"> <div class="card-body"> <h4 class="card-title">Lower Case Pipe</h4> <p ngNonBindable>{{'Welcome to Angular Pipes' | lowercase}}</p> <p>{{'Welcome to Angular Pipes' | lowercase}}</p> </div> </div>
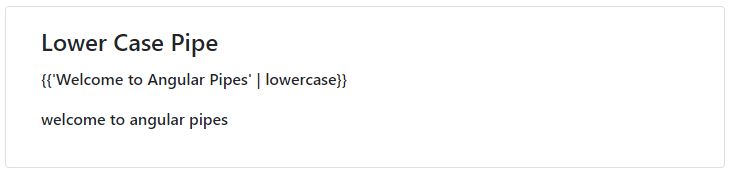
3. TitleCasePipe
As the name suggests, TitleCasePipe transforms text to titlecase.
We can use TitleCasePipe as shown below,
expression | titlecase
here,
expression
is any string valuetitlecase
represent TitleCasePipe
Example :
<div class="card"> <div class="card-body"> <h4 class="card-title">Title Case Pipe</h4> <p ngNonBindable>{{'welcome to angular pipes' | titlecase}}</p> <p>{{'welcome to angular pipes' | titlecase}}</p> </div> </div>
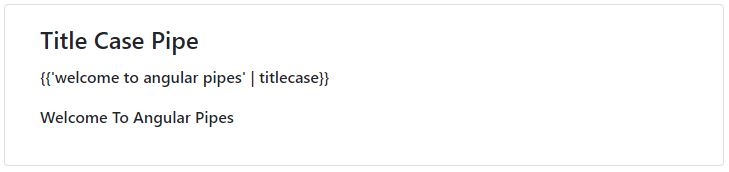
4. DatePipe
DatePipe is very useful to format dates.
We can format any date object or a number (milliseconds since UTC epoch) or an ISO string using DatePipe.
Syntax to use DatePipe is
date_expression | date[:format[:timezone]]
where,
-
date_expression
is a date object or a number (milliseconds since UTC epoch) or an ISO string. format
indicates date-time format. it can be a predefined format as shown below or custom format.'short'
: equivalent to ‘M/d/yy, h:mm a’ (e.g. 6/15/15, 9:03 AM)'medium'
: equivalent to ‘MMM d, y, h:mm:ss a’ (e.g. Jun 15, 2015, 9:03:01 AM)'long'
: equivalent to ‘MMMM d, y, h:mm:ss a z’ (e.g. June 15, 2015 at 9:03:01 AM GMT+1)'full'
: equivalent to ‘EEEE, MMMM d, y, h:mm:ss a zzzz’ (e.g. Monday, June 15, 2015 at 9:03:01 AM GMT+01:00)'shortDate'
: equivalent to ‘M/d/yy’ (e.g. 6/15/15)'mediumDate'
: equivalent to ‘MMM d, y’ (e.g. Jun 15, 2015)'longDate'
: equivalent to ‘MMMM d, y’ (e.g. June 15, 2015)'fullDate'
: equivalent to ‘EEEE, MMMM d, y’ (e.g. Monday, June 15, 2015)'shortTime'
: equivalent to ‘h:mm a’ (e.g. 9:03 AM)
‘mediumTime’: equivalent to ‘h:mm:ss a’ (e.g. 9:03:01 AM)'longTime'
: equivalent to ‘h:mm:ss a z’ (e.g. 9:03:01 AM GMT+1)'fullTime'
: equivalent to ‘h:mm:ss a zzzz’ (e.g. 9:03:01 AM GMT+01:00)
timezone
to be used for formatting. It understands UTC/GMT and the continental US time zone abbreviations, but for general use, use a time zone offset, for example, ‘+0430’ (4 hours, 30 minutes east of the Greenwich meridian) If not specified, the local system timezone of the end-user’s browser will be used.
<div class="card"> <div class="card-body"> <h4 class="card-title">Date Pipe</h4> <p ngNonBindable>{{today | date}}</p> <p>{{today | date}}</p> <hr> <p ngNonBindable>{{today | date:'fullDate'}}</p> <p>{{today | date:'fullDate'}}</p> <hr> <p ngNonBindable>{{today | date:'shortTime'}}</p> <p>{{today | date:'shortTime'}}</p> <hr> <p ngNonBindable>{{today | date:'full'}}</p> <p>{{today | date:'full'}}</p> <hr> <p ngNonBindable>{{today | date:'yyyy-MM-dd HH:mm a z'}}</p> <p>{{today | date:'yyyy-MM-dd HH:mm a z'}}</p> </div> </div>
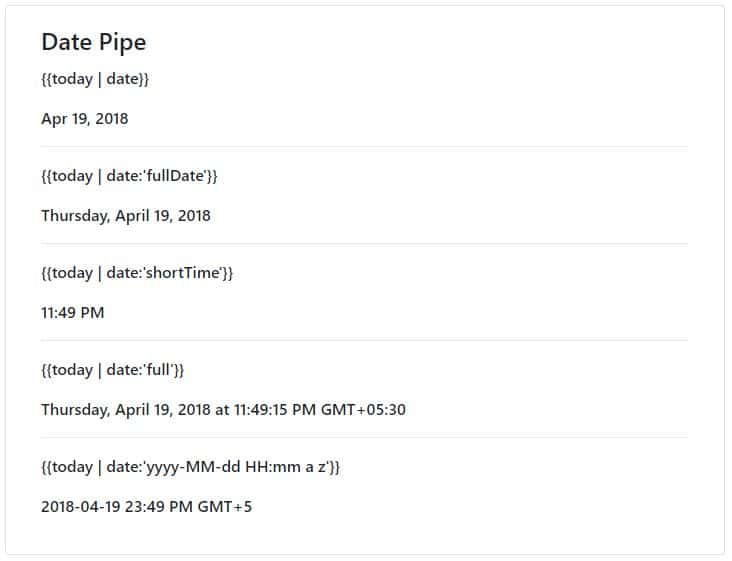
5. DecimalPipe
DecimalPipe used to format numbers into text.
It is used to set the minimum integer digits and minimum-maximum fractional digits.
DecimalPipe also formats numbers into comma separated groups.
Syntax to use DecimalPipe is,
number_expression | number[:digitInfo]
where,
number_expression
is any number variable.- digitInfo is a string with the following format.
{minIntegerDigits}.{minFractionDigits}-{maxFractionDigits}
- where
minIntegerDigits
is the minimum number of integer digits to use. Defaults to 1. minFractionDigits
is the minimum number of digits after fraction. Defaults to 0.maxFractionDigits
is the maximum number of digits after fraction. Defaults to 3.
<div class="card"> <div class="card-body"> <h4 class="card-title">Decimal Pipe</h4> <p ngNonBindable>{{123456.14 | number}}</p> <p>{{123456.14 | number}}</p> <hr> <p ngNonBindable>{{1.3456 | number:'3.1-2'}}</p> <p>{{1.3456 | number:'3.1-2'}}</p> <hr> <p ngNonBindable>{{123456789 | number:'1.2-2'}}</p> <p>{{123456789 | number:'1.2-2'}}</p> <hr> <p ngNonBindable>{{1.2 | number:'4.5-5'}}</p> <p>{{1.2 | number:'4.5-5'}}</p> </div> </div>
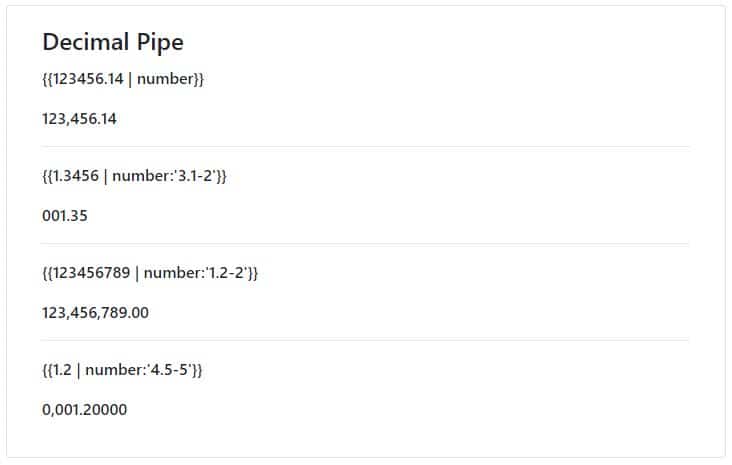
6. CurrencyPipe
CurrencyPipe is very helpful to format number as currency.
It have all the number formatting functionality as DecimalPipe, with additional benefits of assigning currency symbol or code.
Syntax to use CurrencyPipe is,
number_expression | currency[:currencyCode[:display[:digitInfo]]]
where,
number_expression
is any number variable.currency
represent CurrencyPipe.currencyCode
is the ISO 4217 currency code, such as USD for the US dollar and EUR for the euro.display
indicates whether to show the currency symbol or the code.code
: use code (e.g. USD).symbol
(default): use symbol (e.g. $).symbol-narrow
: some countries have two symbols for their currency, one regular and one narrow (e.g. the Canadian dollar CAD has the symbol CA$ and the symbol-narrow $).- boolean (deprecated from v5): true for symbol and false for code If there is no narrow symbol for the chosen currency, the regular symbol will be used.
digitInfo
is a string with the following format.{minIntegerDigits}.{minFractionDigits}-{maxFractionDigits}
- where
minIntegerDigits
is the minimum number of integer digits to use. Defaults to 1. minFractionDigits
is the minimum number of digits after fraction. Defaults to 0.maxFractionDigits
is the maximum number of digits after fraction. Defaults to 3.
<div class="card"> <div class="card-body"> <h4 class="card-title">Currency Pipe</h4> <p ngNonBindable>{{1234567.5555 | currency}}</p> <p>{{1234567.555 | currency}}</p> <hr> <p ngNonBindable>{{1234567 | currency:'INR'}}</p> <p>{{1234567 | currency:'INR'}}</p> <hr> <p ngNonBindable>{{1234567 | currency:'CAD': 'symbol' :'1.2-5'}}</p> <p>{{1234567 | currency:'CAD':'symbol' :'1.2-5'}}</p> <hr> <p ngNonBindable>{{1234567 | currency:'CAD': 'symbol-narrow' :'1.2-5'}}</p> <p>{{1234567 | currency:'CAD':'symbol-narrow' :'1.2-5'}}</p> <hr> <p ngNonBindable>{{1234567.555 | currency:'INR':'symbol':'1.0-0'}}</p> <p>{{1234567.555 | currency:'INR':'symbol':'1.0-0'}}</p> </div> </div>
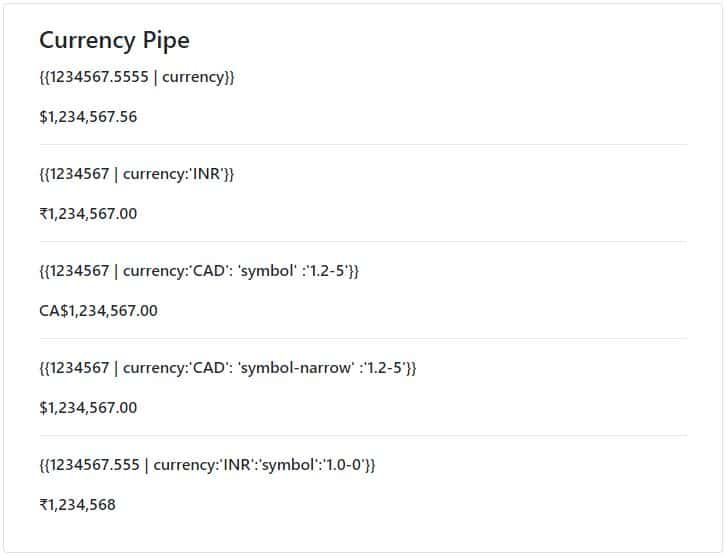
7. PercentPipe
PercentPipe formats number as percentage.
Syntax to use PercentPipe is,
number_expression | percent[:digitInfo]
where,
number_expression
is any number variable.percent
represent PercentPipe.digitInfo
is a string with the following format.{minIntegerDigits}.{minFractionDigits}-{maxFractionDigits}
- where
minIntegerDigits
is the minimum number of integer digits to use. Defaults to 1. minFractionDigits
is the minimum number of digits after fraction. Defaults to 0.maxFractionDigits
is the maximum number of digits after fraction. Defaults to 3.
<div class="card col-md-12"> <div class="card-body"> <h4 class="card-title">Percent Pipe</h4> <p ngNonBindable>{{0.25645 | percent}}</p> <p>{{0.25645 | percent}}</p> <hr> <p ngNonBindable>{{0.25645 | percent:'3.1-5'}}</p> <p>{{0.25645 | percent:'3.1-5'}}</p> <hr> <p ngNonBindable>{{0.25645 | percent:'1.5-5'}}</p> <p>{{0.25645 | percent:'1.5-5'}}</p> </div> </div>
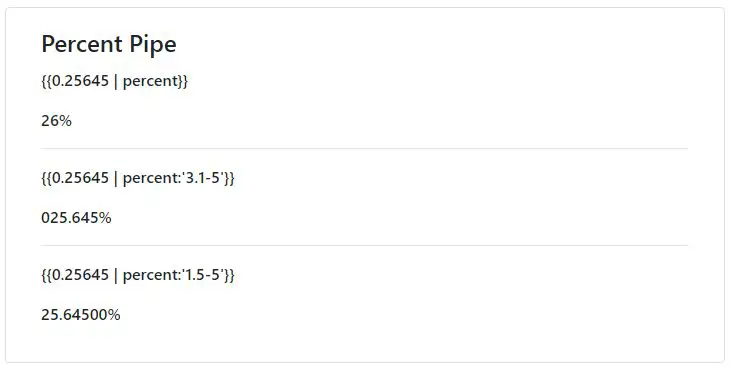
8. SlicePipe
SlicePipe is very useful when you want to slice some part of array or string.
For example, if there is a list of 100 elements and we want to display the 10 elements as pagination is changed, In this situation, we can use SlicePipe, in which start and end parameters of SlicePipe are changed as the page is selected and that sublist of the list is displayed on a screen.
Syntax to use SlicePipe is,
array_or_string_expression | slice:start[:end]
where,
array_or_string_expression
is any array object or string variable.slice
represent SlicePipe.start
: The starting index of the subset to return.a positive integer: return the item at start index and all items after in the list or string expression.
a negative integer: return the item at start index from the end and all items after in the list or string expression.
if positive and greater than the size of the expression: return an empty list or string.
if negative and greater than the size of the expression: return entire list or string.
-
end
: The ending index of the subset to return.- omitted: return all items until the end.
- if positive: return all items before end index of the list or string.
- if negative: return all items before end index from the end of the list or string.
All behavior is based on the expected behavior of the JavaScript API Array.prototype.slice()
and String.prototype.slice()
.
Example :
<div class="card"> <div class="card-body"> <h4 class="card-title">Slice Pipe</h4> <p ngNonBindable>{{[1,2,3,4,5,6] | slice : 2}}</p> <p>{{[1,2,3,4,5,6] | slice : 2}}</p> <hr> <p ngNonBindable>{{[1,2,3,4,5,6] | slice : 2:4}}</p> <p>{{[1,2,3,4,5,6] | slice : 2:4}}</p> <hr> <p ngNonBindable>{{[1,2,3,4,5,6] | slice : 1:-2}}</p> <p>{{[1,2,3,4,5,6] | slice : 1:-2}}</p> <hr> <p ngNonBindable>{{languages | slice : 2:5}}</p> <p>{{languages | slice : 2:5}}</p> <hr> </div> </div>
here, languages
is the string array initialized in a component. as
languages = ['Java','PHP', '.Net','JQuery', 'JavaScript','Angular','AngularJS']
[1,2,3,4,5,6]
is the numeric array used directly in interpolation.
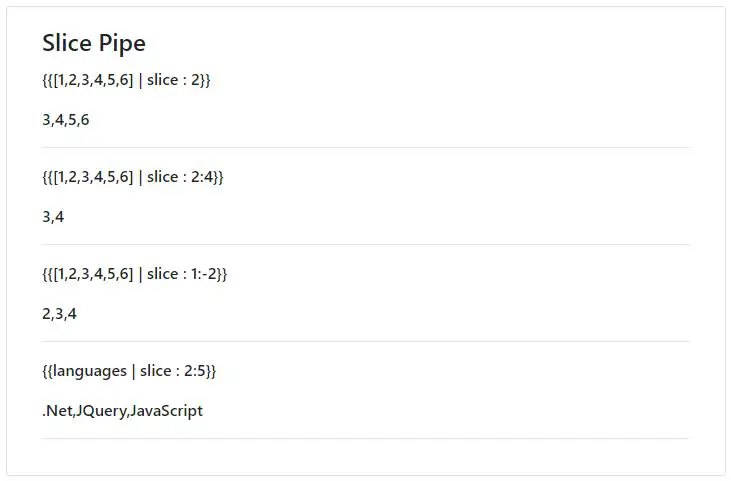
9. JsonPipe
JsonPipe is used to display object in JSON format. It is useful for debugging.
JsonPipe converts a value into a string using JSON.stringify()
.
Syntax to use JsonPipe is,
value | json
where,
value
is any object component.json
represent JsonPipe.
<div class="card"> <div class="card-body"> <h4 class="card-title">Json Pipe</h4> <p ngNonBindable>{{data | json}}</p> <p>{{data | json}}</p> </div> </div>
here, data
is the object initialized in a component.
data = { 'id': 20, 'name': { 'firstname': 'Angular', 'lastname': 'Pipes' } };
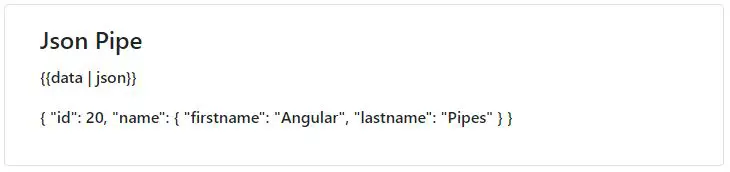
10. AsyncPipe
Generally, If we are getting the result using observable or promise, We need to use following steps,
- Subscribe the observable or promise.
- Wait for a callback.
- Once a result is received, store the result of the callback in a variable.
- the last step is to bind that variable on the template.
Using AsyncPipe
we can use promises and observables directly in our template, without subscribing the observable and storing the result on an intermediate property or variable.
The AsyncPipe
internally subscribes to an Observable or Promise and returns the latest value it has emitted. When a new value is emitted, the AsyncPipe
marks the component to be checked for changes.
When the component gets destroyed, the AsyncPipe
unsubscribes automatically to avoid potential memory leaks.
Syntax to use AsyncPipe is,
observable_or_promise_expression | async
Example :
<div class="card"> <div class="card-body"> <h4 class="card-title">Async Pipe</h4> <p ngNonBindable>{{time | async}}</p> <p>{{time | async}}</p> </div> </div>
here,
-
time
is the observable created in a component.
time = new Observable<string>((observer: Subscriber<string>) => { setInterval(() => observer.next(new Date().toString()), 1000); });
-
async
represent AsyncPipe.
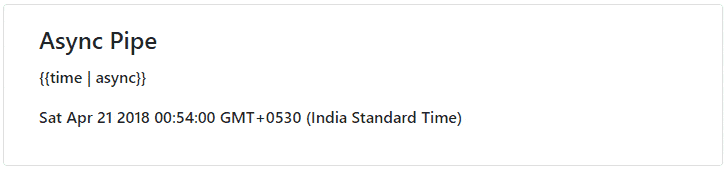
11. I18nSelectPipe
I18nSelectPipe is the generic selector that displays the string that matches the current value.
For example, if we want to replace the text like ‘happy’ with the text-emogie like ‘:-)’, or ‘sad’ with ‘:-(‘, then we can do this using the I18nSelectPipe.
Syntax to use I18nSelectPipe is,
expression | i18nSelect: mapping
here,
-
expression
is value. -
i18nSelect
represent I18nSelectPipe. mapping
is an is an object that indicates the text that should be displayed for different values of the providedexpression
. If none of the keys of the mapping match the value of the expression, then the content of theother
key is returned when present, otherwise an empty string is returned.
<div class="card"> <div class="card-body"> <h4 class="card-title">I18nSelectPipe</h4> <p ngNonBindable>I am {{'happy' | i18nSelect : emogieMap}}</p> <p >I am {{'happy' | i18nSelect : emogieMap}}</p> <hr> <p ngNonBindable>I am {{'sad' | i18nSelect : emogieMap}}</p> <p >I am {{'sad' | i18nSelect : emogieMap}}</p> <hr> <p ngNonBindable>I am {{'none' | i18nSelect : emogieMap}}</p> <p >I am {{'none' | i18nSelect : emogieMap}}</p> </div> </div>
here, emogieMap
is the map created in a component as
emogieMap = {'happy':':-)', 'sad':':-(' ,'other':':-|'};
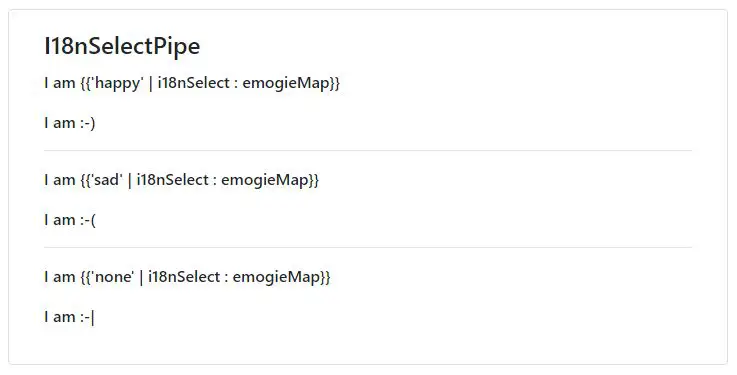
We can chain pipes together in potentially useful combinations.
Syntax to use pipes chain is,
expression | pipe1 | pipe2 | ...
Example :
<div class="card"> <div class="card-body"> <h4 class="card-title">Pipes Chain</h4> <p ngNonBindable>{{time | async | date:'mediumTime'}}</p> <p >{{time | async | date:'mediumTime'}}</p> </div> </div>
here,
time
is the observable (used in AsyncPipe example)async
gives current time at every second.- the result of
time | async
, used as input fordate
pipe, which formats the current date withmediumDate
format
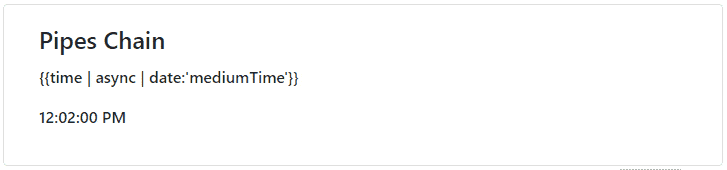
As we have seen above, angular comes with a collection of important pipes. These pipes are very useful to transform text from one form to another form.
As in-built pipes, we can also create own custom pipe.
Let’s see How to create Custom Pipes?
We need to follow these steps to create Custom Pipe. here, I am creating FilterPipe for example.
- Angular Pipes are the class with
@Pipe
decorator. so first create a class in a different file with@Pipe
Decorator.
here, I have createdFilterPipe
class infilter.pipe.ts
file (separate file).import { Pipe } from '@angular/core'; @Pipe({ name: 'filter' }) export class FilterPipe { }
name
in @Pipe metadata is used to represent this pipe on HTML template. - Now implement
PipeTransform
interface from@angular/core
package, which comes withtransform()
abstract method.import { Pipe, PipeTransform } from '@angular/core'; @Pipe({ name: 'filter' }) export class FilterPipe implements PipeTransform { transform(value: any, ...args: any[]) { throw new Error("Method not implemented."); } }
transform()
method has one mandatory parameter, which is the input value, and other optional parameters. we can change this method, as per our requirement. - Now update the
transform()
method :import { Pipe, PipeTransform } from '@angular/core'; @Pipe({ name: 'filter' }) export class FilterPipe implements PipeTransform { transform(array: string[], startWith: string): any { let temp: string[] = []; temp = array.filter(a => a.startsWith(startWith)); return temp; } }
Here I want to filter string from the list, so input is a string array, and
startWith
is one parameter. - Now our pipe is ready to use, but before that, we need to register it in
@NgModule
declarations section.import { FilterPipe } from './filter.pipe'; @NgModule({ declarations: [ ... FilterPipe ], ... }) export class AppModule { }
Let’s use above pipe on a template.
<div class="card"> <div class="card-body"> <h4 class="card-title">Custom Pipe</h4> <div class="form-group"> <input type="text" class="form-control" placeholder="Enter Search String..." #startWith> </div> <ul class="list-group"> <li class="list-group-item" *ngFor="let l of languages | filter : startWith.value">{{l}}</li> </ul> </div> </div>
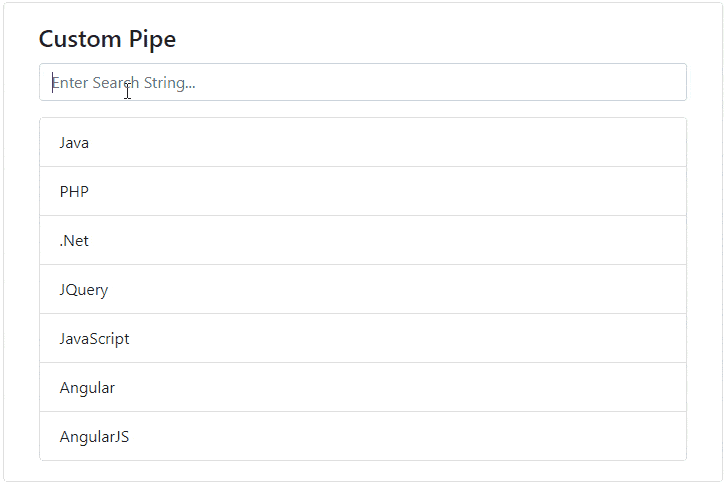
- Note
PipeTransform
is not mandatory to implement. but the transform
method is essential to a pipe. The PipeTransform interface defines that method and guides both tooling and the compiler. Technically, it’s optional; Angular looks for and executes the transform method regardless.
- Tip
One more faster way to create Custom Angular Pipes is using Angular CLI
Use below Angular CLI command to create a pipe.
ng g pipe <pipe-name>
this command generates Pipe with transform()
method, and register it in @NgModule
. We just need to update transform method for specific logic.
In this chapter, we have seen
- What is the use of Angular Pipes ?
- Built-in Pipes
- Pipes Chain
- Steps to create Custom Pipe with Example.