PDFMake is a popular javascript library for client-side and server-side pdf generation. In my previous article Angular Export to PDF using PDFMake we have seen PDFMake setup and pdf generation with online resume builder application.
PDFMake provides features like insert an image, QR Code, Table Of Contents, Header and Footers, SVG, List and so on.
PDFMake supports insert image in PDF, but one drawback of is It only supports adding an image from the local file system or in the form of a base64 data URL. It doesn’t support external URL directly.
So, In this article we will see How to insert image from URL in PDF using PDFMake ?
As we discussed, PDFMake directly does not support the insert image from URL. It supports base64 format or image path from the local file system. but in client-side pdf generation you have only one option, insert an image as base64 format.
So here we will implement it with the below approach :
- Get base64 formatted data URL from the external image URL.
- Add base64 formatted data URL in document definition.
- Generate PDF
We will write a function that will generateBase64 formatted Data URL from External Image URL.
getBase64ImageFromURL(url) { return new Promise((resolve, reject) => { var img = new Image(); img.setAttribute("crossOrigin", "anonymous"); img.onload = () => { var canvas = document.createElement("canvas"); canvas.width = img.width; canvas.height = img.height; var ctx = canvas.getContext("2d"); ctx.drawImage(img, 0, 0); var dataURL = canvas.toDataURL("image/png"); resolve(dataURL); }; img.onerror = error => { reject(error); }; img.src = url; }); }
Source code of the above function is from the StackOverflow answer, I have changed it to return promise.
Above function returns promise
so that we can use it with async/await
while generating document definition.
We will create one async
function, which will configure the document definition and generate the PDF.
async showPdf() { let docDefinition = { content: [ { text: 'PDF Generated with Image from external URL', fontSize : 20 }, { image: await this.getBase64ImageFromURL( "https://images.pexels.com/photos/209640/pexels-photo-209640.jpeg?auto=compress&cs=tinysrgb&dpr=1&w=300" ) } ] }; pdfMake.createPdf(docDefinition).open(); }
As you can see, we are passing external URL in getBase64ImageFromURL
function which will generate base64 data URL, and add in document definition.
Here we are using async/await
, We have to wait to generate pdf until document definition is built with base64 image.
The
await
expression causesasync
function execution to pause until aPromise
is settled, that is fulfilled or rejected, and to resume execution of theasync
function after fulfillment. When resumed, the value of theawait
expression is that of the fulfilled Promise.
after that, we will call the showPdf()
function. it uses to open pdf pdfMake.createPdf(docDefinition).open();
This will generate pdf as below :
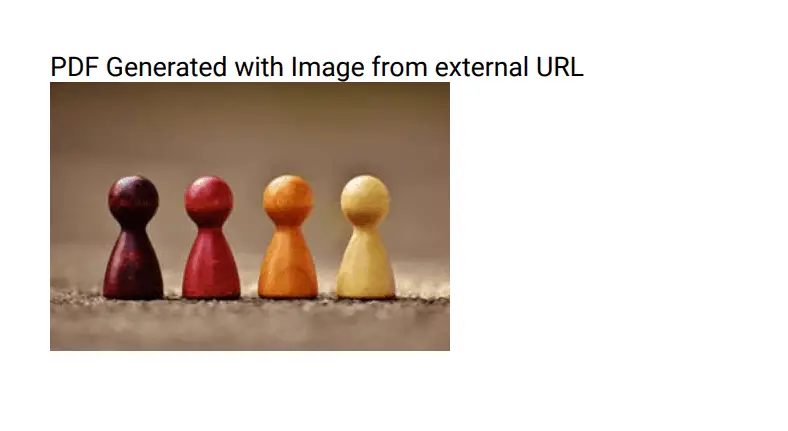
- Note
Using the above approach we can generate pdf with an image from URL.
This approach is good if you don’t have to add so many images, but if your pdf includes so many images from URL then above approach is might be not a good solution, because for each image you have to generate data URL and add it in document definition, which will affect the application performance.
In this article, we have seen insert an image from a URL in PDF using PDFMake.
I hope you like this article, please provide your valuable feedback and suggestions in below comment section🙂.
For more updates, Follow us 👍 on NgDevelop Facebook page.